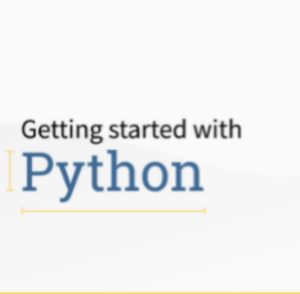
Python-Getting Started Training
Duration : 3 Days
Date : 22, 23, 24 May 2023
Overview
The training program gets participants started with the basics of python and ensures that they have a good understanding of all popular and standard concepts needed to start working on Python immediately. The participants undergoing the course will have a good understanding of – Basic Python Programming and its various constructs, commonly used Python libraries, Input & Outputs, Error Handling, etc
Prerequisite for Python
Good proficiency in other programming langaugs like C/C++/Java/PHP etc
This covers Basic Python concepts that can be covered in 3 days. This covers Python data types, operators, conditions, for, while loop, functions, seq and non sequential objects, reading and writing to file command line.
Course Contents
Python Background
- Background on Python
- Introduction to Machine Learning
- Why Python is used in Machine Learning?
- Details of Python 1, 2, 3
- Structuring with Indentation
- 2 Modes: Normal and Interactive (Shell)
- Why Python is so popular for ML
- Python Success Stories – Apps in Python
- Python Basics: indent, blocks, CMD
- Dynamic typing and strongly typed
Tools/IDE Intro
- Demo on CMD
- Jupyter Intro/Pycharm IDE
Structure and Type
- Basic Structure of Python program
- Jupyter Tutorial
- In class Demo on Python
- Primary Data types – int, float, …
- Demo on size of int, float
- Demo on casting
- Demo on complex
- Coding tasks
Python Operators & Conditional Statements
- Operators
- Operators and their precedence
- Arithmetic, comparison, membership, assignment, Logical, bitwise, identity
- id (Understand how objects are stored in memory)
- Demo on variables and address
- Conditional Statements
- Membership
- In class Demo in and is
- In class Demo Operators, priority, conditional
- Demo on bitwise
- Coding Tasks
Loops
- While Loops
- For Loops
- How for loop in python differs from Java
- For loop written in While using iterable *
- Coding Tasks
Functions
- Functions and different types of functions
- Calling protocol for mutable and immutable
- Positional, keyword, default, dynamic, lambda
- Demo on lambda Function
- Assignment on Pub entry validator
Print and Reading Writing from Terminal
- Output with Print
- Formatted output
- input and raw_input via the keyboard
- Reading and writing from terminals
- Demo on Reading and Writing from Terminal
- Assignment on Reading from terminal
Sequential and Non Sequential DT
- Sequential Data Types – String, List, Tuple,
- byte_sequences, byte arrays, range object
- Common functions across all sequential types
- [], +, +=, *, *=, len, in, count, slicing
- Mutable and Immutable
String
- String Operations
- Access, +,*, slice
- split, join
- Other string functions
- Demo on String and its functions
- Demo on String slice
- Demo on String Format
Lists
- List
- List type explained
- No arrays in basic python
- Contents of list
- List inside a list, tuple inside a list, set, dict
- Slicing works in list also
- Functions in list – Creation,….
- Demo on list slice
- Assignment on List access
- Assignment on List slice and duplicates
Tuple
- Tuple
- Tuple Type explained – immutable
- Functions
- Can a tuple contain a list
- Why tuple
- Demo on Tuple
- Demo on Tuple and address
- Demo on Tuple immutability
- Coding Tasks
Byte, Byte Array, Range
- Byte, Byte Sequence Byte Array
- range object
- Demo on Byte, Byte Sequence, Range
Non Sequential
- Non Sequential Dictionaries, Sets
Dictionary
- Dictionaries: Concepts, and why
- Key Value concept
- simple eg of dict
- iterators -keys, values, both
- check for keys using in
- check for values using in
- Functions in dict
- what can come as key? Any immutable object
- What can come as values: Any mutable or immutable object
- Demo on dict creation, iteration and its functions
- Demo: Can dicts be indexed even though it is non-sequential?
- Coding Tasks on dict
Set
- Set Concept
- Mutable
- Functions in Set
- Immutable
- Demo on set and its functions
- Coding Tasks on set – Build a Probability based Sentiment analyzer
Modular Programming
- Modular Programming and Modules
- Intro to modules, packages, Numpy intro, Sklearn Intro (* Depending on time)
Persistence
- Pickle – Brief intro (Details in Advanced)