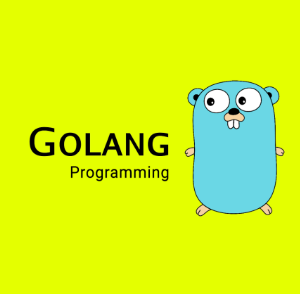
Go Programming Online Workshop
Duration : 3 Days (9 AM to 5 PM)
Date : 18,19, 20 November 2020
Overview
Go Programming workshop provides highly intensive hands-on training on both Go programming language and the Go ecosystem, which is packed with real-world guidance using code examples. This course lets the audience build real-world backend systems and Microservices using the Go ecosystem. Attendees can dive into production systems after completing this training. This course will also provide architecture guidance for building scalable backend systems in Go.
Prerequisites:
There are no prerequisites for this training program however a knowledge of any other programming language is helpful.
Course Contents
The Go Language, Control Structures
Why Go?
The Beginnings of Go
- Go vs Other languages
- Supported Platforms, Cross Compiling
- Key Distinguishing Features
Setting Up Go
- Downloading and Installing Go Setting up Go
- Environment Variables
- Why do we need Git, Mercurial, etc.?
- Go Playground
Basic Program, Go Tools
- Hello World, packages, import, main & main
- Go build
- Go run
Working with Strings
- String Functions
- String Formatting
Variables and Assignment
- Variable differences new
- Multiple assignment
- Values
- Variables
- Constants
Errors
- Errors in Go
- Error Conventions
- Custom Errors panic and recover
- Defer
Cross Compiling
- Cross Compiling Go Programs
Functions
- Writing a Function
- Return Values
- Multiple Return Values
Pointers, Parameters, Return Values
- Pointers
- Parameters
- Pass by Value, Pass by Reference
Control Statements
- If/Else
- Switch
Arrays, Slices, Maps, For
- For
- Arrays Slices
- Maps
- Range , continue, break, goto, fallthrough
“OOP” – Structs, Interfaces, Encapsulation, Inheritance, Polymorphism
Structs
- Structs
- Struct Members
- Anonymous Struct Members
- Methods on Structs
- Pointer and Value Receivables in struct methods
- How structs take place of objects
Interfaces
- Interfaces
Encapsulation, “Object Hierarchy”, “OOP”
- Data Hiding
- Struct Inheritance with Composition
- Polymorphism
Workspaces, go get, and managing dependencies
- Workspace Directory Structure go get command
- go env variables and library search
- vendor Directory dep
Concurrency
Concurrency: Goroutines, Parallelism
- Concurrency with goroutines
- Concurrency and Parallelism
Concurrency: Handling Race Conditions
- Example of Race Condition
Concurrency: SyncGroup, Wait, Mutexes
- Sync, Wait
- Mutexes
- Deadlocks with Mutexes
- RW Mutexes
Concurrency: Channels
- Channel Direction
- Closing Channels
- Range Over Channels
- Channels – Select Timeouts
Concurrency: Canceling and Context
- Leaky Goroutines
- Canceling Goroutines
- Canceling with Context
- Canceling with Deadline/Timeout
- Closing a Context Sends Done() to All
- Closing a Context Sends Done() to All Derived Contexts
Web Programming Templates
- How to Use Templates
- Structs and Templates
- Text Templates
- HTML Templates
JSON
- Struct to JSON
- Marshaling and Unmarshaling
Regular Expressions
- Using regex in Go http package
- Running a Web Server and Handling Requests
- HTTP Return Codes
- Regex Routes
- Variables
- Serving Static Files
- Context gorilla package Installing gorilla mux
- Routing URLs
- Sub routers
REST
- What is REST?
- CRUD and REST?
- HTTP Requests and REST
- A REST Project in Go
Files, Databases, Testing and Benchmarking, Logging
Files
- Reading Files
- Writing Files
- Reading and Writing to any pipe
Databases
- init and then main importing to register with init sql package
- working with oracle
- working with cassandra
Unit Testing
- Writing and Running Unit Tests
- Table driven tests go cover
Benchmarking
- What are benchmarks?
- Writing and Running Benchmarks
- Additional libraries for testing
Profiling & Optimisation
- Walkthrough of Profiling Example
Logging and Debugging
- Standard Library Logging
- SysLog logrus vscode and Delve
- Delve Command Line
- Logging Filenames, Functions, and Line numbers
Monitoring Go Applications Using New Relic
- track transactions, outbound requests, database calls, and other parts of Go application’s behavior and provides a running overview of garbage collection, goroutine activity, and memory use.
- github.com/newrelic/go-agent
Distributed Tracing Techniques Using Zipkin
- Tracing Spans
- Tracing UI
- Reporting
- https://github.com/openzipkin/zipkin-go
API Documentation Using Swagger
- Requirements
- serialize and deserialize
- Code Commenting & representation of RESTful API.
- https://github.com/go-swagger/go-swagger